Handling Empty Results Using ContentUnavailableView in SwiftUI
Published on Oct 1, 2024Get a quick glimpse of how Tiny Currency simplifies currency conversion with up to date rates, multi-currency support, and easy-to-use widgets. Perfect for on-the-go use, our app ensures you're always prepared, no matter where your travels take you.
📱 iOS 17.0+
In iOS 17, Apple added a new ContentUnavailableView
to SwiftUI. This view helps with showing an empty state for any use case.
Usage
There is a handy built in use case for Search content. Let's take a look at an example:
struct ContentView: View {
@State var searchText = ""
@State var searchResults = [String]()
var body: some View {
NavigationStack {
List {
ForEach(searchResults, id: \.self) { item in
NavigationLink {
Text(item)
} label: {
Text(item)
}
}
}
.navigationTitle("Search Example")
.searchable(text: $searchText)
.overlay {
if searchResults.isEmpty {
ContentUnavailableView.search(text: searchText)
}
}
}
}
}
In the example above, we see a simple searchable view, and if the search results are empty, the ContentUnavailableView is shown.
In the example, I've used the variante that accepts text, but there is also ContentUnvailableView.search
for if you don't want to display the text either.
This is what they both look like:
![]() | ![]() |
But you can also build your own custom version as well!
The ContentUnavailableView
is in fact a SwiftUI view, so we can build one like so:
ContentUnavailableView(
"Oops! 😢",
systemImage: "questionmark.diamond",
description: Text("We didn't find anything...")
)
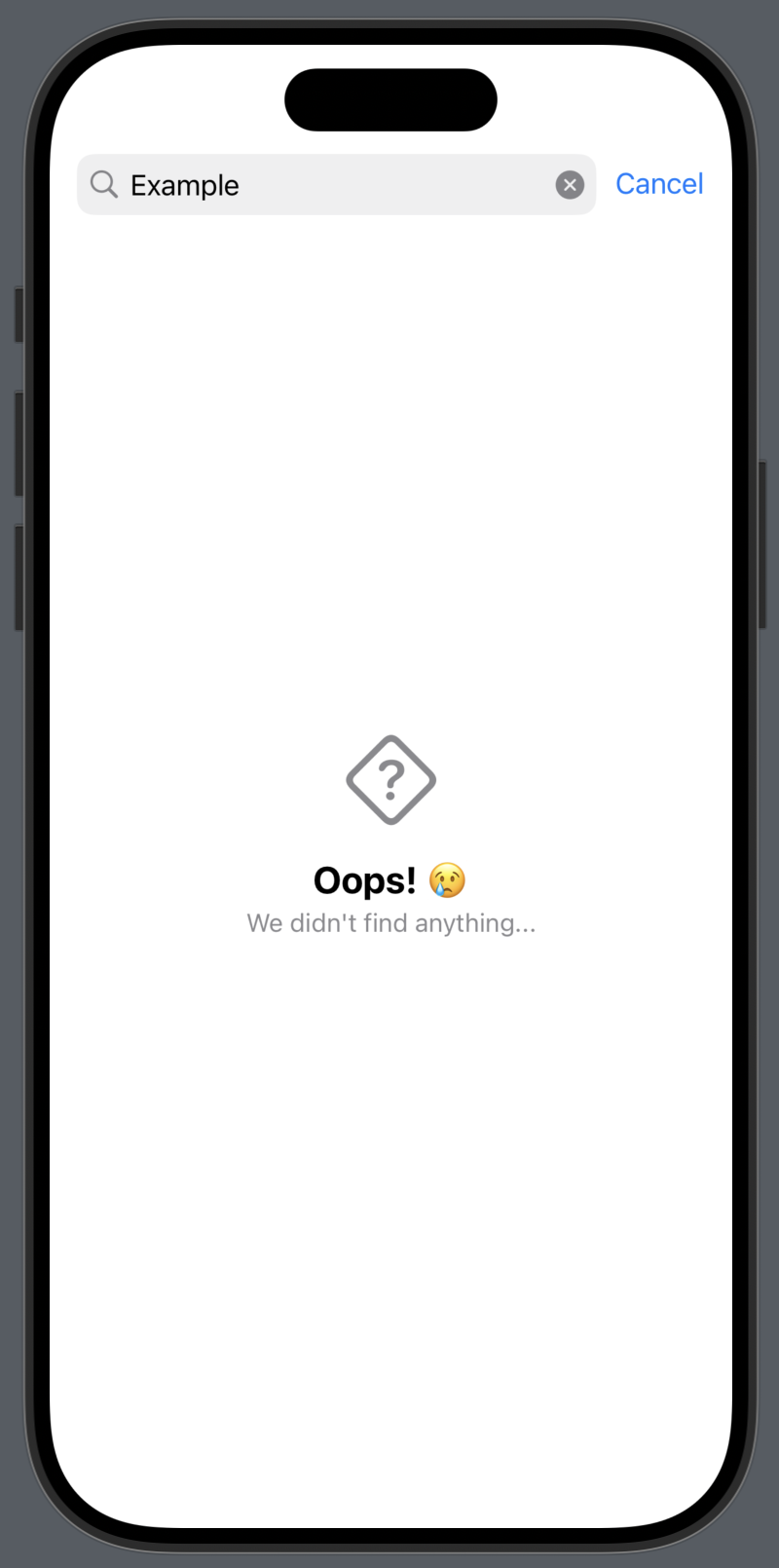
There you have it! A simple view that can showcase empty states easily. There might even be other use cases for displaying this than when content is unavailable, but the naming does also provide some developer intent in what it's use case is.